Today In this tutorial, I will discuss a very easy way to create a complete user registration and login system using PHP and MySQL.
Read this article until the end and do not skill a line for good understanding. If you are new to programming than also do not worry, here you will get a very easy tutorial to create user register and login system using PHP and MySQL.
Before we start actual coding first we have to install the software in our system.
How to Install XAMPP Server in Windows and Ubuntu?
Windows:
To download XAMPP Server in Windows Operating System click on the link given below and download the XAMPP Server form it's the official website.
And follow the instructions given the video below to install XAMPP Server in Windows.
To start XAMPP Server starts Apache and MySQL.
http://localhost/phpmyadmin/
Step 2: Make the XAMPP package executable
Open the terminal in your system and execute the following command:
Step 1: Create the database and table as the structure given below.
Step 2: Create a file signup.php and paste the code given below.
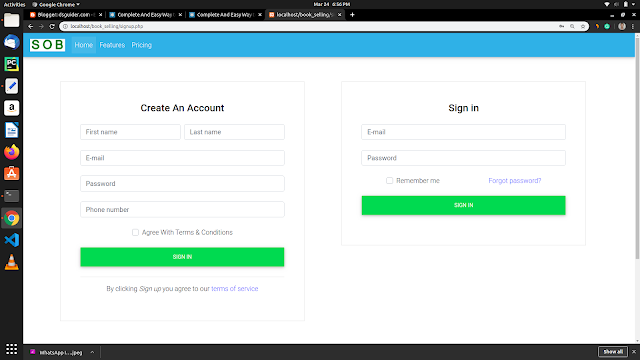
- After that load PHPMyAdmin in your browser.
http://localhost/phpmyadmin/
![]() |
Start PHPMyAdmin in your browser |
Ubuntu:
To install XAMPP Server in Ubuntu follow the steps given below:
Step 1: Download the XAMPP package
Visit the official website download XAMPP installation package for Linux: Click Here
Open the terminal in your system and execute the following command:
$ cd /home/[username]/Downloads
$ chmod 755 xampp-linux-x64-7.2.10-0-installer.run
$ ls -l xampp-linux-x64-7.2.10-0-installer.run
sudo ./xampp-linux-7.2.10-0-installer.run
Step 3: To start Xampp Server in your Ubuntu system execute the following command
$ sudo /opt/lampp/lampp start
How to create a Registration and Login system using PHP and MySQL?
First, we design a Registration form with the help of HTML, CSS, and JAVASCRIPT.
Here to design Registration form, we will use the Bootstrap front end library.Step 1: Create the database and table as the structure given below.
![]() |
Structure of the Database |
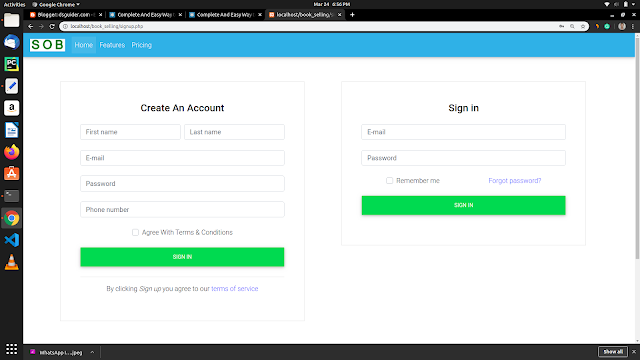
<?php
session_start();
include('include/config.php');
$errors = array();
if (isset($_POST['submit'])){
$fname = mysqli_real_escape_string($conn, $_POST['fname']);
$lname = mysqli_real_escape_string($conn, $_POST['lname']);
$email = mysqli_real_escape_string($conn, $_POST['email']);
$mobile = mysqli_real_escape_string($conn, $_POST['mobileno']);
$pass = mysqli_real_escape_string($conn, $_POST['password']);
$user_check_query = "SELECT * FROM users WHERE email = '$email' or mobile = '$mobile' LIMIT 1";
$result = mysqli_query($conn, $user_check_query);
$user = mysqli_fetch_assoc($result);
if($user){//user already exist
if($user['email']==$email){
array_push($errors, "User Already Exist!");
}
if($user[mobile]==$mobile){
array_push($errors, 'User Already Exist!');
}
}
else{
$p = md5($pass);
$query = "INSERT INTO users(fname, lname, email, mobile, password) VALUES ('$fname', '$lname', '$email', '$mobile', '$p')";
mysqli_query($conn, $query);
$_SESSION['user'] = $email;
header('location: welcome.php');
}
}
if(isset($_POST['login'])){
$email = mysqli_real_escape_string($conn, $_POST['email']);
$pas = mysqli_real_escape_string($conn, $_POST['password']);
$pas = md5($pas);
$query = "SELECT * FROM users WHERE email='$email' and password='$pas'";
$result = mysqli_query($conn, $query);
if(mysqli_num_rows($result)==1){
$_SESSION['user'] = $email;
header('location: index.php');
}
else {
array_push($errors, "Wrong username/password combination");
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title></title>
<!-- Font Awesome -->
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css">
<!-- Google Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap">
<!-- Bootstrap core CSS -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.4.1/css/bootstrap.min.css" rel="stylesheet">
<!-- Material Design Bootstrap -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mdbootstrap/4.14.0/css/mdb.min.css" rel="stylesheet">
<!-- JQuery -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<!-- Bootstrap tooltips -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.4/umd/popper.min.js"></script>
<!-- Bootstrap core JavaScript -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.4.1/js/bootstrap.min.js"></script>
<!-- MDB core JavaScript -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/mdbootstrap/4.14.0/js/mdb.min.js"></script>
<!---CSS Include--->
<link rel="stylesheet" href="style/main.css">
</head>
<body>
<!----Header Navigation Bar--->
<?php include('include/header1.php'); ?>
<!---Login and Signup form--->
<div class="section">
<div class="signup frm">
<!-- Default form register -->
<?php if (count($errors) > 0) : ?>
<div class="alert alert-danger alert-dismissible fade show" role="alert">
<?php foreach ($errors as $error) : ?>
<?php echo $error ?>
<?php endforeach ?>
<button type="button" class="close" data-dismiss="alert" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<?php endif ?>
<form class="text-center border border-light p-5" action="signup.php" method="post">
<p class="h4 mb-4">Create An Account</p>
<div class="form-row mb-4">
<div class="col">
<!-- First name -->
<input type="text" id="defaultRegisterFormFirstName" class="form-control" placeholder="First name" name="fname" required>
</div>
<div class="col">
<!-- Last name -->
<input type="text" id="defaultRegisterFormLastName" class="form-control" placeholder="Last name" name="lname" required>
</div>
</div>
<!-- E-mail -->
<input type="email" id="defaultRegisterFormEmail" class="form-control mb-4" placeholder="E-mail" name="email" required>
<!-- Password -->
<input type="password" id="defaultRegisterFormPassword" class="form-control" placeholder="Password" aria-describedby="defaultRegisterFormPasswordHelpBlock" name="password" required>
<br>
<!-- Phone number -->
<input type="text" id="defaultRegisterPhonePassword" class="form-control" placeholder="Phone number" aria-describedby="defaultRegisterFormPhoneHelpBlock" name="mobileno" required>
<!---Location--->
<br>
<!-- Newsletter -->
<div class="custom-control custom-checkbox">
<input type="checkbox" class="custom-control-input" id="defaultRegisterFormNewsletter">
<label class="custom-control-label" for="defaultRegisterFormNewsletter">Agree With Terms & Conditions</label>
</div>
<!-- Sign up button -->
<button class="btn btn-success my-4 btn-block" type="submit" name="submit">Sign in</button>
<!-- Social register -->
<hr>
<!-- Terms of service -->
<p>By clicking
<em>Sign up</em> you agree to our
<a href="" target="_blank">terms of service</a>
</form>
<!-- Default form register -->
</div>
<!--login form--->
<div class="login frm">
<!-- Default form login -->
<form class="text-center border border-light p-5" action="signup.php" method='post'>
<p class="h4 mb-4">Sign in</p>
<!-- Email -->
<input type="email" id="defaultLoginFormEmail" class="form-control mb-4" placeholder="E-mail" name='email' required>
<!-- Password -->
<input type="password" id="defaultLoginFormPassword" class="form-control mb-4" placeholder="Password" name='password' required>
<div class="d-flex justify-content-around">
<div>
<!-- Remember me -->
<div class="custom-control custom-checkbox">
<input type="checkbox" class="custom-control-input" id="defaultLoginFormRemember">
<label class="custom-control-label" for="defaultLoginFormRemember">Remember me</label>
</div>
</div>
<div>
<!-- Forgot password -->
<a href="">Forgot password?</a>
</div>
</div>
<!-- Sign in button -->
<button class="btn btn-success btn-block my-4" type="submit" name='login'>Sign in</button>
</form>
<!-- Default form login -->
</div>
</div>
<!---Footer starts from here--->
<div class="foot">
<?php include('include/footer.php'); ?>
</div>
</body>
</html>